Selenium WebDriver Example / Sample test application - Learn Selenium / Learn WebDriver
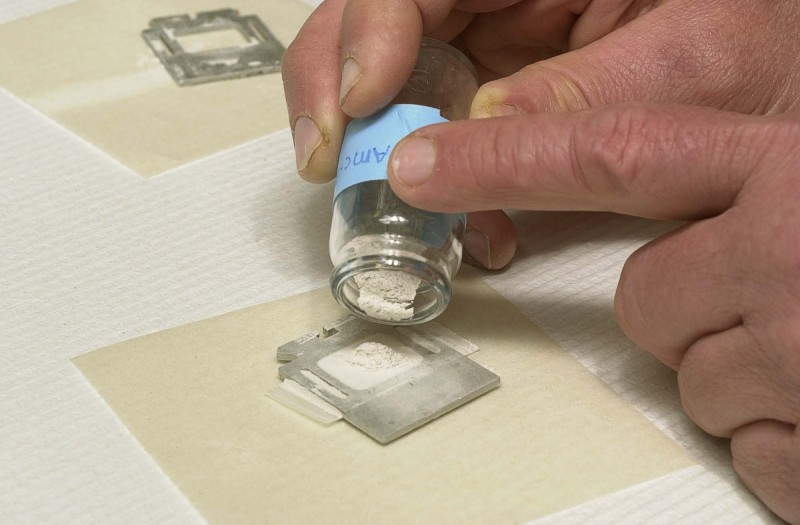
For all the newbies who are trying to learn selenium there are very few examples except for this one.
If you already know how to configure eclipse and webdriver then you are reading the right post.
For those who want some info on configuring eclipse - check this earlier post.
The best thing I could think of was automating my own blog as an example.
Here are the steps:
- Launch the browser
- Navigate to http://go-gaga-over-testing.blogspot.in
- In the customized google search field type "Selenium"
- Click on search
- Validate the search results are displayed
- Close the browser
Create a new class under that
Now you can either record using selenium IDE and Export the test case as JUnit 4 (WebDriver) and save the file as
as a "".java or use any tool (Six tools for Selenium Object Identification) to get the object properties to directly use in the webdriver test case.So we are all set to start coding
This is how the raw test case would look like:
package testSelenium;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import java.util.concurrent.TimeUnit;
import static junit.framework.Assert.assertTrue;
public class FirstClass {
private WebDriver driver;
@Before
public void setUp() throws Exception {
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
}
@After
public void tearDown() throws Exception {
driver.quit();
}
@Test
public void testSearchExportedFromIDE() throws Exception {
String baseUrl = "http://go-gaga-over-testing.blogspot.in/";
driver.get(baseUrl);
WebElement searchField = driver.findElement(By.name("search"));
//searchField.clear();
searchField.sendKeys("Selenium");
searchField.submit();
WebElement searchResult = driver.findElement(By.className("gs-visibleUrl"));
String expected = "http://go-gaga-over-testing.blogspot.com/";
String actual = searchResult.getText();
assertTrue(actual.contains(expected));
}
}
It needs some cleaning up. Note that it is good enough to be executed but this is just a starting point.
You can play around by changing the properties and using other identification types
Check this page for more information and to understand and learn selenium.
A few things that can be changed:
1. Firefox Profile should be the default profile
2. While debugging you will need a lot of message boxes so just add this snippet
//import javax.swing.JOptionPane; //JOptionPane.showMessageDialog(null,actual );
3. Take screenshots on failures
The test case can be further transformed into something that uses page object model and that may be easier to read.
The Page Object pattern represents the screens of your web app as a series of objects - Read this!
Something more readable + PO model would look like this:
package testSelenium;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxProfile;
import org.openqa.selenium.firefox.internal.ProfilesIni;
import static junit.framework.Assert.assertTrue;
public class FirstClass {
private WebDriver driver;
@Before
public void setUp() throws Exception {
ProfilesIni profile = new ProfilesIni();
FirefoxProfile ffprofile = profile.getProfile("default");
driver = new FirefoxDriver(ffprofile);
}
@After
public void tearDown() throws Exception {
driver.quit();
}
@Test
public void testSearchExportedFromIDE() throws Exception {
Homepage home = new Homepage(driver);
SearchResultPage searchResult = home.searchFor("Selenium");
String expected = http://go-gaga-over-testing.blogspot.com/;
String actual = searchResult.getHeadLine();
//JOptionPane.showMessageDialog(null,actual );
assertTrue(actual.contains(expected));
}
}
This needs two support classes, therefore add two new classes:
package testSelenium;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import java.io.File;
import java.io.IOException;
public class Homepage {
private WebDriver driver;
public Homepage(WebDriver driver) {
this.driver = driver;
String baseUrl = "http://go-gaga-over-testing.blogspot.in";
driver.get(baseUrl + "/");
}
public SearchResultPage searchFor(String location) {
try {
WebElement searchField = driver.findElement(By.name("search"));
searchField.sendKeys(location);
searchField.submit();
} catch (RuntimeException e) {
takeScreenShot(e, "searchError");
}
return new SearchResultPage(driver);
}
private void takeScreenShot(RuntimeException e, String fileName) {
File screenShot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
try {
FileUtils.copyFile(screenShot, new File(fileName + ".png"));
} catch (IOException ioe) {
throw new RuntimeException(ioe.getMessage(), ioe);
}
throw e;
}
}
AND
package testSelenium;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
public class SearchResultPage {
private WebDriver driver;
public SearchResultPage(WebDriver driver) {
this.driver = driver;
if (!driver.getTitle().contains("Go gaga over testing")) {
throw new IllegalStateException("This is not Go gaga over testing: " + driver.getCurrentUrl());
}
}
public String getHeadLine() {
WebElement searchResult = driver.findElement(By.className("gs-visibleUrl"));
return searchResult.getText();
}
If you are successful doing this with any of your web pages. You are now ready to dive in deep , start by reading all these documents and practicing a lot!
Happy Learning!
http://code.google.com/p/selenium/wiki/NextSteps
http://code.google.com/p/selenium/wiki/PageObjects
Comments
Post a Comment